The landscape of browser testing has traditionally been dominated by tools such as Selenium which incorporate a real web browser (e.g., Chromium) and orchestrate prepared tasks against a target test site. This differs from other popular load testing frameworks such as JMeter where the basic HTTP requests are sent and the responses directly analyzed. Browser testing confers the advantage of interacting with a website in the same way that a user would. This is achieved by rendering the page in real-time, including dynamic elements and scripting. With the introduction of k6 and its extensible modules, browser testing is possible in a similar manner as Chromium through the browser
module. In this brief post, we will compare k6 to Selenium to highlight the strengths and differences between these two frameworks.
The k6 Browser Module
One of the core principles of k6 rests in its extensibility with both built-in and third-party modules. The module we will focus on is the browser
module which exposes interactivity with a Chromium browser client. As mentioned earlier, this allows k6 to access and render web pages in the same manner a real user would using a web browser application.
Some essential examples of the core capabilities that the browser
module exposes from the official k6 documentation include:
browser.context()
– Returns the current browser contextbrowser.isConnected
– Indicates if the connection to the browser process is activebrowser.newContext()
– Creates and returns a new browser contextbrowser.newPage()
– Creates a new page in a new browser contextbrowser.version()
– Returns the browser application version
Calling these methods allows your k6 test to simulate requests and render interactive pages. In the examples below, we will illustrate how to assemble these attributes and function calls to easily create a basic browser test.
Running Browser Tests with k6
There are several good examples containing the basic functionality of a k6 browser test in the official browser
module documentation pages. We can start with a basic example that can be easily extended to suit your particular test needs:
import { browser } from 'k6/experimental/browser';
export const options = {
scenarios: {
ui: {
executor: 'shared-iterations',
options: {
browser: {
type: 'chromium',
},
},
},
},
}
export default async function () {
const page = browser.newPage();
try {
await page.goto('https://test.k6.io/');
page.screenshot({ path: 'screenshots/screenshot.png' });
} finally {
page.close();
}
}
In this example, the browser
module is imported, and the newPage()
function is used to open and render that page. Using the page.screenshot()
function, a screenshot image is saved to a .jpg
file before the session is closed. The url in await page.goto()
can be modified to contain the address to any website. To experiment with this example yourself, simply save the above code to a .js
file and start a new k6 load test on RedLine13. You can download the output files to see the result.
Interactive k6 Browser Test Example
In another example which you can also find on the k6 documentation pages illustrates how to interact with elements on the page to perform a useful task:
import { browser } from 'k6/experimental/browser';
export const options = {
scenarios: {
ui: {
executor: 'shared-iterations',
options: {
browser: {
type: 'chromium',
},
},
},
},
}
export default async function () {
const page = browser.newPage();
try {
await page.goto('https://test.k6.io/my_messages.php');
// Enter login credentials
page.locator('input[name="login"]').type('admin');
page.locator('input[name="password"]').type('123');
page.screenshot({ path: 'screenshots/screenshot.png' });
} finally {
page.close();
}
}
In this case, a user log is simulated to a page containing a form where a login and password is entered. This example is hosted by k6 here, allowing you to directly use this example and experiment with k6 testing capabilities. Successful submission of the form yields the following result:
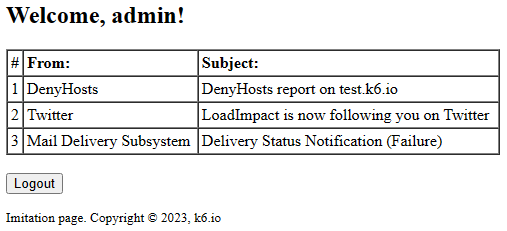
Comparing k6 Browser Tests with Selenium
Selenium offers a suite of browser testing tools, but perhaps the most comparable is Selenium WebDriver. Both are scripted frameworks, however the scripting language of WebDriver derives from Java (versus k6 which uses JavaScript). This translates to a different ecosystem of libraries and extensibility, though the concept is very similar. The following is an example of a basic script provided in the official Selenium documentation pages:
package dev.selenium.getting_started;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.time.Duration;
public class FirstScript {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://www.selenium.dev/selenium/web/web-form.html");
driver.getTitle();
driver.manage().timeouts().implicitlyWait(Duration.ofMillis(500));
WebElement textBox = driver.findElement(By.name("my-text"));
WebElement submitButton = driver.findElement(By.cssSelector("button"));
textBox.sendKeys("Selenium");
submitButton.click();
WebElement message = driver.findElement(By.id("message"));
message.getText();
driver.quit();
}
}
This example is one of the official examples that Selenium provides from WebDriver, and along with other similar examples can be found in their GitHub repository. The above script as executed will load the specified target page, enter information into a named text field, and submit a form. This is similar functionality to the second test provided above for k6. In comparing the two, k6 offers more abstraction and completes the similar action in less lines of code. This is partially a consequence of the differences between the two scripting languages used, and partially due to design decisions for concise code in k6.
Which Framework to Use
Both k6 using the browser
module, and Selenium WebDriver perform similar tasks. They both use a scripting language to control a real browser instance (such as Chromium). The decision to use one framework versus the other will come down to several factors. Language familiarity is one consideration, with k6 using JavaScript and WebDriver being based on Java. It can also be argued that k6 affords more agility in its more concise code. Conversely, WebDriver leverages capabilities and dependencies which may be unique to Java. k6 is also a newer technology which continues to evolve and garner community support. The final decision between each framework ultimately rests on the unique requirements of your test plan.
Did you know that RedLine13 offers a full-featured, time-limited free trial? Sign up now, and move your k6 tests into the cloud with RedLine13 today!