When writing your k6 test scripts, there are certain common tasks that are recurrently encountered. In this post, we will discuss examples on how to accomplish several of these common tasks. Most of these examples are illustrated in the official k6 documentation pages.
Entering Data into Web Forms
User submission forms are a common target test endpoint. Tests such as these must be designed in a way that form fields are identified and mapped to useful values prior to submission. In k6, we can use the submitForm()
method on a Response
object. This comes as part of the k6 JavaScript API, and can be implemented in your test as follows:
response.submitForm({ formSelector: 'login-form', fields: { username: 'test_user', password: 'test_password' } })
HTTP Basic Authentication
A relatively simple yet essential task, basic authentication over HTTP allows your test to access a secured endpoint by providing a username and password. The most straightforward approach to this is using an interpolated string to inject credentials into your URLs. In the example below, we are using two variables, username
and password
to create a credentials string that is incorporated into the fully formed request:
import http from 'k6/http'; const credentials = `${username}:${password}`; const url = `https://${credentials}@www.your-domain.com/endpoint/`; let response = http.get(url);
Data Parameterization from JSON
As your test plan grows with sophistication, commonly there is a need to apply a dataset to your test. Some examples include such things as iterating over a list of user accounts, or a similar list of variables to submit to an API or a web form. One way that k6 natively supports this is through loading a dataset from a locally stored JSON file:
import { SharedArray } from 'k6/data'; const data = new SharedArray('some data name', function () { return JSON.parse(open('./data.json')).users; });
This loads a JSON file into a JavaScript collection. For illustrative purposes, here is an example that k6 provides in their official documentation:
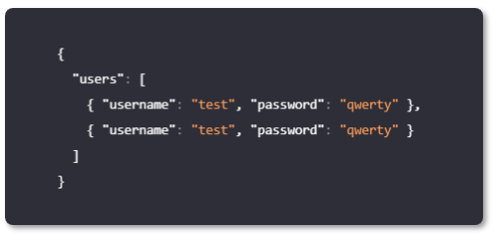
To access fields, we can call properties on the data
object (which is an instance of a SharedArray
object) that we created in the previous step:
export default function () { console.log(data[0].username); }
Note that data
becomes an indexed collection, with property accessors mapped to the field names specified in the JSON data file.
Data Parameterization from CSV
Similar to the above example which references data in a locally stored JSON file, you can load a dataset from a CSV file. It should be noted however that k6 lacks native support for CSV file parsing. Thankfully due to its extensibility, there is a third-party extension PapaParse that can be leveraged to extend this capability:
import papaparse from 'https://jslib.k6.io/papaparse/5.1.1/index.js'; import { SharedArray } from 'k6/data'; const data = new SharedArray('CSV data', function () { return papaparse.parse(open('./data.csv'), { header: true }).data; });
You can access and use the data in the same manner as the JSON example above, through the SharedArray
object. In this case, the data
object as an indexed collection extends property accessors mapped to the column names in the CSV file header row.
Dynamic Setting of Request Parameters
Though it is possible to manually construct URLs for requests as strings, there are a few considerations we must pay attention to. One especially relates to URL encoding of special characters. However, k6 does offer the means to abstract parameter setting of URLs through a convenient extension:
import { URL } from 'https://jslib.k6.io/url/1.0.0/index.js'; import http from 'k6/http'; const url = new URL('https://www.your-domain.com/product'); url.searchParams.append('item_code', 'A101'); url.searchParams.append('item_quantity', '15'); url.searchParams.append('colors', ['red', 'green']); const res = http.get(url.toString());
The URLSearchParams
object can be used to construct a fully qualified URL string by substituting variable names and associated values. It automatically handles delimiters and special characters, and as shown in the example above, can also handle collections.
Did you know that RedLine13 offers a full-featured, time-limited free trial? Sign up now, and start testing today!